EDITオブジェクトのパラメーターの定義・設定方法
ファイルの新規作成時に表の位置などを定義
この記事では、「EDITオブジェクト」のツールを作りながら、データを集計する方法を解説します。
参考記事:EDITオブジェクトを用いて曜日ごとの集計表を描画する方法
EDITオブジェクトはもともとMT5専用のオブジェクトです。MT4では、バージョンアップしていく中で使うことが可能となりました。四角形と文字を組み合わせたテキストボックスのようなもので、プログラムからしか作成できないオブジェクトです。今回は、月曜日~金曜日の陽線、陰線、十字線の数をカウントして、その集計結果を表で描画してみましょう。集計範囲は指定した「年」の数値とし、その年の指定はチャート上のEDITオブジェクトで変更可能とします。
まず新規作成で「UpDownTable_DOW」というファイルを作り、表の位置などをパラメーターで最初から定義しておきます。
EDITオブジェクトを表示するX位置(横の位置)は「POSX」、初期値を「0」、Y位置(縦の位置)は「POSY」、初期値を「15」、年と曜日を並べるタイトルの列の幅は「WIDTH_TITLE」、初期値を「80」、陽線・陰線・十字線といったデータを並べる列の幅は「WIDTH_DATA」、初期値を「150」、高さは「HEIGHT」、初期値を「30」に設定します。曜日等を並べるタイトル1列、データ3列(陽線・陰線・十字線)の全4列という構成です。
今回は「OnChartEvent」を使うので、「カスタムインディケータのイベントハンドラ」で「OnChartEvent」のみにチェックを入れます。これでファイルの準備は完了です。
EDITのパラメーターを設定
EDITオブジェクトのサンプルコードは、MQL4リファレンスから拝借します。MQL4リファレンスの目次にある「Constants, Enumerations and Structures」→「Objects Constants」→「Object Types」をクリックすると、オブジェクトの一覧が表示されるので、その中から「OBJ_EDIT」を選択し、あらかじめ用意されている下記コードをコピーして「ChartEvent function」 の下に貼り付けます。
//+------------------------------------------------------------------+
//| Create Edit object |
//+------------------------------------------------------------------+
bool EditCreate(const long chart_ID=0, // chart's ID
const string name="Edit", // object name
const int sub_window=0, // subwindow index
const int x=0, // X coordinate
const int y=0, // Y coordinate
const int width=50, // width
const int height=18, // height
const string text="Text", // text
const string font="Arial", // font
const int font_size=10, // font size
const ENUM_ALIGN_MODE align=ALIGN_CENTER, // alignment type
const bool read_only=false, // ability to edit
const ENUM_BASE_CORNER corner=CORNER_LEFT_UPPER, // chart corner for anchoring
const color clr=clrBlack, // text color
const color back_clr=clrWhite, // background color
const color border_clr=clrNONE, // border color
const bool back=false, // in the background
const bool selection=false, // highlight to move
const bool hidden=true, // hidden in the object list
const long z_order=0) // priority for mouse click
{
//--- reset the error value
ResetLastError();
//--- create edit field
if(!ObjectCreate(chart_ID,name,OBJ_EDIT,sub_window,0,0))
{
Print(__FUNCTION__,
": failed to create \"Edit\" object! Error code = ",GetLastError());
return(false);
}
//--- set object coordinates
ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,x);
ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,y);
//--- set object size
ObjectSetInteger(chart_ID,name,OBJPROP_XSIZE,width);
ObjectSetInteger(chart_ID,name,OBJPROP_YSIZE,height);
//--- set the text
ObjectSetString(chart_ID,name,OBJPROP_TEXT,text);
//--- set text font
ObjectSetString(chart_ID,name,OBJPROP_FONT,font);
//--- set font size
ObjectSetInteger(chart_ID,name,OBJPROP_FONTSIZE,font_size);
//--- set the type of text alignment in the object
ObjectSetInteger(chart_ID,name,OBJPROP_ALIGN,align);
//--- enable (true) or cancel (false) read-only mode
ObjectSetInteger(chart_ID,name,OBJPROP_READONLY,read_only);
//--- set the chart's corner, relative to which object coordinates are defined
ObjectSetInteger(chart_ID,name,OBJPROP_CORNER,corner);
//--- set text color
ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr);
//--- set background color
ObjectSetInteger(chart_ID,name,OBJPROP_BGCOLOR,back_clr);
//--- set border color
ObjectSetInteger(chart_ID,name,OBJPROP_BORDER_COLOR,border_clr);
//--- display in the foreground (false) or background (true)
ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back);
//--- enable (true) or disable (false) the mode of moving the label by mouse
ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection);
ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection);
//--- hide (true) or display (false) graphical object name in the object list
ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden);
//--- set the priority for receiving the event of a mouse click in the chart
ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order);
//--- successful execution
return(true);
}
パラメーターの並びに関しては、設定したいものを前の方に、初期設定のまま使うものを後ろの方に移動させると分かりやすいです。それと同時に、今回はフォントサイズを「14」、フォントを「メイリオ」、文字色を「白」、背景色を「黒」、ラインの色を「白」に変更します。これでパラメーターの設定は完了です。
bool EditCreate(string name = "Edit", // object name
const int x = 0, // X coordinate
const int y = 0, // Y coordinate
const int width = 50, // width
const int height = 18, // height
const string text = "Text", // text
const bool read_only = true, // ability to edit
const color clr = clrWhite, // text color
const color back_clr = clrBlack, // background color
const color border_clr = clrWhite, // border color
const long chart_ID = 0, // chart's ID
const int sub_window = 0, // subwindow index
const string font = "メイリオ", // font
const int font_size = 14, // font size
const ENUM_ALIGN_MODE align = ALIGN_CENTER, // alignment type
const ENUM_BASE_CORNER corner = CORNER_LEFT_UPPER, // chart corner for anchoring
const bool back = false, // in the background
const bool selection = false, // highlight to move
const bool hidden = true, // hidden in the object list
const long z_order = 0) // priority for mouse click
本記事の監修者・HT FX
2013年にFXを開始し、その後専業トレーダーへ。2014年からMT4/MT5のカスタムインジケーターの開発に取り組む。ブログでは100本を超えるインジケーターを無料公開。投資スタイルは自作の秒足インジケーターを利用したスキャルピング。
EA(自動売買)を学びたい方へオススメコンテンツ
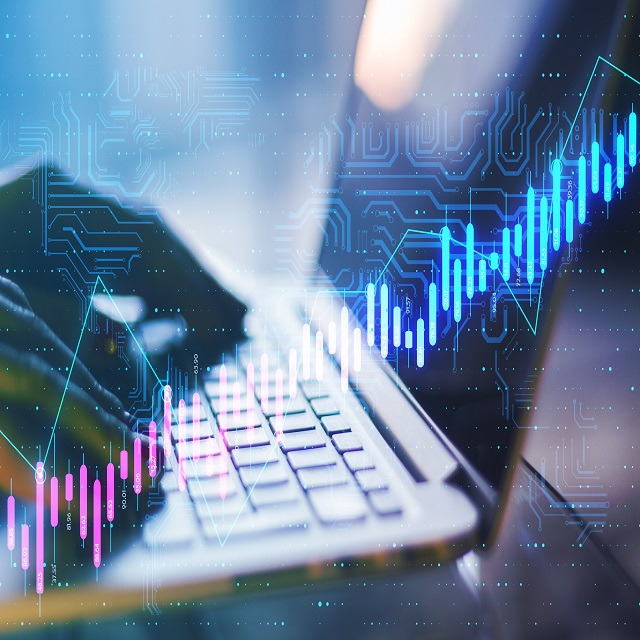
OANDAではEA(自動売買)を稼働するプラットフォームMT4/MT5の基本的な使い方について、画像や動画付きで詳しく解説しています。MT4/MT5のインストールからEAの設定方法までを詳しく解説しているので、初心者の方でもスムーズにEA運用を始めることが可能です。またOANDAの口座をお持ちであれば、独自開発したオリジナルインジケーターを無料で利用することもできます。EA運用をお考えであれば、ぜひ口座開設をご検討ください。
本ホームページに掲載されている事項は、投資判断の参考となる情報の提供を目的としたものであり、投資の勧誘を目的としたものではありません。投資方針、投資タイミング等は、ご自身の責任において判断してください。本サービスの情報に基づいて行った取引のいかなる損失についても、当社は一切の責を負いかねますのでご了承ください。また、当社は、当該情報の正確性および完全性を保証または約束するものでなく、今後、予告なしに内容を変更または廃止する場合があります。なお、当該情報の欠落・誤謬等につきましてもその責を負いかねますのでご了承ください。